リストの項目を削除する
リストの項目を削除する場合は、1つずつ消す方法と、範囲を指定してまとめて消す方法と、全部まとめて消す方法があります。
まずは項目を1つ指定して削除する方法です。DefaultListModelクラスで用意されている"remove"メソッドを使います。
remove public Object remove(int index)
リスト内の指定された位置の要素を削除し、リストから削除された要素を返し ます。 インデックスが範囲 (index < 0 || index >= size()) 外の場合は ArrayIndexOutOfBoundsException をスローします。 パラメータ: index - 削除される要素のインデックス
引数に削除したい項目のインデックス番号を指定します。
次に範囲を指定して削除する方法です。DefaultListModelクラスで用意されている"removeRange"メソッドを使います。
removeRange public void removeRange(int fromIndex, int toIndex)
指定されたインデックス範囲にあるコンポーネントを削除します。削除対象は 上限と下限の値を含むので、1 と 5 の範囲を指定すると、インデックス 1 と 5 のコンポーネント、そしてその間のすべてのコンポーネントが削除されます。 インデックスが無効な場合は ArrayIndexOutOfBoundsException をスローしま す。fromIndex > toIndex の場合は IllegalArgumentException をスローし ます。 パラメータ: fromIndex - 範囲の下限のインデックス toIndex - 範囲の上限のインデックス
引数に削除したい項目の範囲として、開始インデックスと終了インデックスを指定します。
最後に全ての項目を削除する方法です。DefaultListModelクラスで用意されている"clear"メソッドを使います。
clear public void clear()
リストからすべての要素を削除します。リストは、この呼び出しが返されると、 呼び出しが例外をスローしないかぎり、空になります。
ではサンプルプログラムで試してみましょう。
import javax.swing.*; import java.awt.*; import java.util.Vector; import java.awt.event.*; import javax.swing.event.*; public class JListSample extends JFrame implements ActionListener{ protected JList list; protected DefaultListModel model; public static void main(String[] args){ JListSample test = new JListSample("JListSample"); /* 終了処理を変更 */ test.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); test.setBounds( 10, 10, 250, 160); test.setVisible(true); } JListSample(String title){ setTitle(title); model = new DefaultListModel(); /* 初期データをモデルに追加する */ StringBuffer sb; for (int i = 0 ; i < 15 ; i++){ sb = new StringBuffer(); sb.append("項目"); sb.append(i); model.addElement(new String(sb)); } list = new JList(model); JScrollPane sp = new JScrollPane(); sp.getViewport().setView(list); sp.setPreferredSize(new Dimension(200, 80)); JPanel p = new JPanel(); p.add(sp); getContentPane().add(p, BorderLayout.CENTER); JButton removeButton = new JButton("Remove"); removeButton.addActionListener(this); removeButton.setActionCommand("removeButton"); JButton rangeButton = new JButton("Range"); rangeButton.addActionListener(this); rangeButton.setActionCommand("rangeButton"); JButton clearButton = new JButton("Clear"); clearButton.addActionListener(this); clearButton.setActionCommand("clearButton"); JPanel p2 = new JPanel(); p2.add(removeButton); p2.add(rangeButton); p2.add(clearButton); getContentPane().add(p2, BorderLayout.SOUTH); } public void actionPerformed(ActionEvent e){ String actionCommand = e.getActionCommand(); if (actionCommand.equals("removeButton")){ if (!list.isSelectionEmpty()){ int index = list.getSelectedIndex(); model.remove(index); } }else if (actionCommand.equals("rangeButton")){ if (!list.isSelectionEmpty()){ int minIndex = list.getMinSelectionIndex(); int maxIndex = list.getMaxSelectionIndex(); model.removeRange(minIndex, maxIndex); } }else if (actionCommand.equals("clearButton")){ model.clear(); }else{ return; } } }
実行結果は下記のようになります。
項目を1つ選択します。
この状態でRemoveボタンを押すと下記のようになります。
次に範囲を指定して削除するために項目を2つ選択します。
この状態でRangeボタンを押すと下記のようになります。
最後にClearボタンを押すと全ての項目が削除されます。
( Written by Tatsuo Ikura )
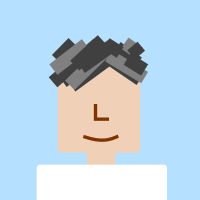
著者 / TATSUO IKURA
プログラミングや開発環境構築の解説サイトを運営しています。