タブを削除する
広告
追加されたタブを削除する方法を確認します。タブを削除するにはJTabbpedPaneクラスで用意されている「remove」メソッドを使います。
remove public void remove(int index)
指定されたインデックスに対応するタブとコンポーネントを削除します。 パラメータ: index - tabbedpane から削除するコンポーネントのインデックス 例外: IndexOutOfBoundsException - インデックスが範囲外の場合 (index < 0 || index >= タブの総数)
1番目の引数に削除したいタブのインデックスを指定します。インデックスはタブが追加された順に割り振られた番号で最初のタブのインデックスは「0」となります。存在しないインデックスを指定すると例外が発生します。
実際の使い方は次のようになります。
JTabbedPane tabbedpane = new JTabbedPane(); tabbedpane.addTab("title1", new JButton("button1")); tabbedpane.addTab("title2", new JButton("button2")); tabbedpane.remove(0);
全てのタブを削除する
現在追加されているタブを全て削除する方法を確認します。全てのタブを削除するにはJTabbpedPaneクラスで用意されている「removeAll」メソッドを使います。
removeAll public void removeAll()
tabbedpane からすべてのタブおよび対応するコンポーネントを削除します。
このメソッドを実行すると全てのタブが削除されます。
実際の使い方は次のようになります。
JTabbedPane tabbedpane = new JTabbedPane(); tabbedpane.addTab("title1", new JButton("button1")); tabbedpane.addTab("title2", new JButton("button2")); tabbedpane.removeAll();
サンプルプログラム
では簡単なサンプルを作成して試してみます。
import javax.swing.*; import java.awt.BorderLayout; import java.awt.event.*; public class JTabbedPaneTest3 extends JFrame implements ActionListener{ JTabbedPane tabbedpane; public static void main(String[] args){ JTabbedPaneTest3 frame = new JTabbedPaneTest3(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setBounds(10, 10, 300, 200); frame.setTitle("タイトル"); frame.setVisible(true); } JTabbedPaneTest3(){ tabbedpane = new JTabbedPane(); JPanel tabPanel1 = new JPanel(); tabPanel1.add(new JButton("button1")); JPanel tabPanel2 = new JPanel(); tabPanel2.add(new JLabel("Name:")); tabPanel2.add(new JTextField("", 10)); JPanel tabPanel3 = new JPanel(); tabPanel3.add(new JButton("button2")); tabbedpane.addTab("tab1", tabPanel1); tabbedpane.addTab("tab2", tabPanel2); tabbedpane.addTab("tab3", tabPanel3); JPanel buttonPanel = new JPanel(); JButton deleteButton = new JButton("delete"); deleteButton.addActionListener(this); buttonPanel.add(deleteButton); getContentPane().add(tabbedpane, BorderLayout.CENTER); getContentPane().add(buttonPanel, BorderLayout.PAGE_END); } public void actionPerformed(ActionEvent e){ int index = tabbedpane.getSelectedIndex(); if (index != -1){ tabbedpane.remove(index); } } }
上記をコンパイルした後で実行すると次のように表示されます。
画面下部に表示されているボタンをクリックすると、現在選択されているタブを削除します。
( Written by Tatsuo Ikura )
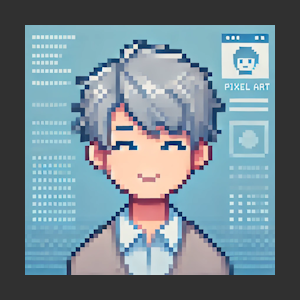
著者 / TATSUO IKURA
これから IT 関連の知識を学ばれる方を対象に、色々な言語でのプログラミング方法や関連する技術、開発環境構築などに関する解説サイトを運営しています。