日本語パラメータの対応(setCharacterEncoding)
日本語が含まれるパラメータを処理する方法として「setCharacterEncoding」メソッドを使う方法を確認します。「HttpServletRequest」インターフェースの親インターフェースの「ServletRequest」インターフェースで定義されています。
もともと文字化けする原因が、パラメータとして送られてきたバイト列から文字列に変換する時に、文字コードとして「ISO-8859-1(Latin1)」を使ってしまうのが問題でした。そこで正しい文字コードをサーブレットに事前に設定が出来れば文字化けは発生しません。「setCharacterEncoding」メソッドはリクエストボディに含まれるデータの文字コードを指定した値に書き換えるメソッドです。
setCharacterEncoding public void setCharacterEncoding(java.lang.String env) throws java.io.UnsupportedEncodingException
Overrides the name of the character encoding used in the body of this request. This method must be called prior to reading request parameters or reading input using getReader(). Parameters: env - a String containing the name of the character encoding. Throws: java.io.UnsupportedEncodingException - if this is not a valid encoding
例えば次のように使います。
public class Sample extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException{ request.setCharacterEncoding("Shift_JIS"); String val = request.getParameter("name"); } }
極めてスマートに文字化けが回避出来るのですが、このメソッドではリクエストボディに含まれるデータの文字コードしか設定できません。「POST」メソッドを使った場合にはクライアントから送られてくるパラメータはリクエストボディに含まれるので問題ありませんが、「GET」メソッドを使った場合にはパラメータはURIの部分に含まれています。その為「setCharacterEncoding」メソッドを使ってもパラメータの文字コードの設定は行えませんので注意してください。
サンプルプログラム
では簡単なサンプルで試して見ます。
フォームが含まれるHTMLページは、送信方法を「POST」にしたものを使います。
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html lang="ja"> <head> <meta http-equiv="Content-Type" Content="text/html;charset=Shift_JIS"> <title>フォームサンプル</title> </head> <body> <p>アンケート調査です</p> <form action="/sample/RequestSample3" method="post"> <table> <tr> <td>氏名</td> <td><input type="text" size="20" value="" name="name"></td> </tr> <tr> <td>年齢</td> <td><input type="text" size="5" value="" name="old"></td> </tr> <tr> <td>好きな果物</td> <td> <select name="food" size="3" multiple> <option value="りんご">りんご</option> <option value="メロン">メロン</option> <option value="ぶどう">ぶどう</option> </select> </td> </tr> </table> <input type="submit" name="button1" value="送信"> </form> </body> </html>
次にフォームから送られてくるリクエストパラメータを処理するサーブレットを作成します。今度は「doGet」メソッドではなく「doPost」メソッドを使います。
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; public class RequestSample3 extends HttpServlet { public void doPost(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException{ response.setContentType("text/html;charset=Shift_JIS"); PrintWriter out = response.getWriter(); request.setCharacterEncoding("Shift_JIS"); String tmp; String name = ""; tmp = request.getParameter("name"); if (tmp == null || tmp.length() == 0){ name = "未設定です"; }else{ name = tmp; } int old; tmp = request.getParameter("old"); if (tmp == null || tmp.length() == 0){ old = -1; }else{ try{ old = Integer.parseInt(tmp); }catch (NumberFormatException e){ old = -1; } } String tmps[] = request.getParameterValues("food"); String food = ""; if (tmps != null){ for (int i = 0 ; i < tmps.length ; i++){ food += tmps[i]; food += " "; } }else{ food = "ありません"; } StringBuffer sb = new StringBuffer(); sb.append("<html>"); sb.append("<head>"); sb.append("<title>サンプル</title>"); sb.append("</head>"); sb.append("<body>"); sb.append("<p>お名前は "); sb.append(name); sb.append(" です</p>"); sb.append("<p>年齢は "); if (old == -1){ sb.append("未設定です</p>"); }else{ sb.append(old); sb.append(" です</p>"); } sb.append("<p>好きな果物は "); sb.append(food); sb.append("です</p>"); sb.append("</body>"); sb.append("</html>"); out.println(new String(sb)); out.close(); } }
サンプルプログラムをコンパイルして作成した「RequestSample3.class」ファイルを別途作成した「web.xml」ファイルを次のように配置します。
D:\ -- servlet-sample | +-- (formsample3.html) | +-- WEB-INF | +-- (web.xml) | +-- classes | +-- (RequestSample3.class)
web.xmlファイルは次のようになります。
<?xml version="1.0" encoding="ISO-8859-1"?> <web-app xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd" version="2.4"> <servlet> <servlet-name>RequestSample3</servlet-name> <servlet-class>RequestSample3</servlet-class> </servlet> <servlet-mapping> <servlet-name>RequestSample3</servlet-name> <url-pattern>/RequestSample3</url-pattern> </servlet-mapping> </web-app>
コンテキストファイルを作成し「(Tomcatをインストールしたディレクトリ)\Tomcat 5.5\conf\Catalina\localhost\」ディレクトリに「sample.xml」ファイルとして保存します。内容は以下の通りです。
<Context path="/sample" docBase="d:/servlet-sample/sample"> </Context>
準備は以上です。ではTomcatを再起動してから「http://localhost:8080/sample/formsample3.html」へブラウザでアクセスして下さい。
フォームが表示されますので、適当に値を入力してから送信ボタンをクリックして下さい。すると次のようにリクエストパラメータの値を取得して表示します。
「POST」メソッドを使うようにすれば簡潔に記述が行えます。
( Written by Tatsuo Ikura )
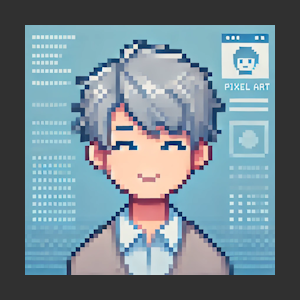
著者 / TATSUO IKURA
これから IT 関連の知識を学ばれる方を対象に、色々な言語でのプログラミング方法や関連する技術、開発環境構築などに関する解説サイトを運営しています。