パスワードフィールドに入力されたテキストを取得する
パスワードフィールドに利用者が入力したテキストを取得する方法について解説します。
(2022 年 04 月 11 日公開 / 2022 年 04 月 11 日更新)
入力されたテキストを取得する
パスワードフィールドに入力された値を取得するには JPasswordField クラスで定義されている getPassword メソッドを使います。
public char[] getPassword()
このTextComponentに格納されたテキストを返します。 ベースとなるドキュメントがnullの場合は、NullPointerExceptionを返します。 セキュリティ強化のために、返された文字の配列を使用したあとは、各文字をゼロに設定してクリアすることをお勧めします。
戻り値:
テキスト
パスワードフィールドに入力された値を char 型の配列として返します。( String クラスのオブジェクトではないので注意して下さい)。
実際の使い方は次のようになります。
JPasswordField pass = new JPasswordField(); char[] password = pass.getPassword();
なお char 型の配列を String クラスのオブジェクトにするには次のように記述します。
JPasswordField pass = new JPasswordField(); char[] password = pass.getPassword(); String passwordstr = new String(password);
他の文字列と比較したい場合などはいったんStringクラスのオブジェクトに変換して下さい。
それでは簡単なサンプルプログラムを作って試してみます。テキストエディタで次のように記述したあと、 JSample2_1.java という名前で保存します。
import javax.swing.JFrame; import javax.swing.JPasswordField; import javax.swing.JPanel; import javax.swing.JLabel; import javax.swing.JButton; import javax.swing.JOptionPane; import java.awt.Container; import java.awt.BorderLayout; import java.awt.event.ActionListener; import java.awt.event.ActionEvent; class JSample2_1 extends JFrame implements ActionListener{ JPasswordField pass; public static void main(String args[]){ JSample2_1 frame = new JSample2_1("MyTitle"); frame.setVisible(true); } JSample2_1(String title){ setTitle(title); setBounds(100, 100, 600, 400); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JLabel label = new JLabel("Enter your password"); pass = new JPasswordField(30); JButton button = new JButton("Check"); button.addActionListener(this); JPanel p = new JPanel(); p.add(label); p.add(pass); p.add(button); Container contentPane = getContentPane(); contentPane.add(p, BorderLayout.CENTER); } public void actionPerformed(ActionEvent e){ String password = new String(pass.getPassword()); if (password.equals("abcd")){ JLabel label = new JLabel("Success"); JOptionPane.showMessageDialog(this, label, "Success", JOptionPane.INFORMATION_MESSAGE); }else{ JLabel label = new JLabel("Failure"); JOptionPane.showMessageDialog(this, label, "Failure", JOptionPane.ERROR_MESSAGE); pass.setText(""); } } }
次のようにコンパイルを行います。
javac JSample2_1.java
コンパイルが終わりましたら実行します。
java JSample2_1
ラベル、パスワードフィールド、ボタンを追加してあります。
パスワードフィールドにパスワードを入力しボタンをクリックします。間違ったパスワードの場合はダイアログに "Failure" と表示されます。
正しいパスワードの場合はダイアログに "Success" と表示されます。
-- --
パスワードフィールドに利用者が入力したテキストを取得する方法について解説しました。
( Written by Tatsuo Ikura )
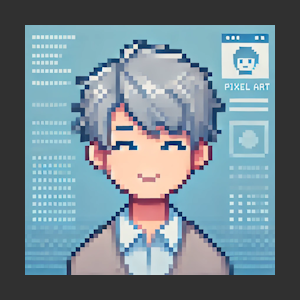
著者 / TATSUO IKURA
これから IT 関連の知識を学ばれる方を対象に、色々な言語でのプログラミング方法や関連する技術、開発環境構築などに関する解説サイトを運営しています。