ステータスコードの設定(setStatus)
クライアントにレスポンスを返す際に、ステータスコードを設定しなかった場合にはデフォルトで「SC_OK」が設定されます。正常なデータを返す場合にはこのままで構いませんが他のステータスコードをクライアントに返す方法を確認します。
ステータスコードを設定するには「HttpServletResponse」インターフェースで定義されている「setStatus」メソッドを使います。
setStatus public void setStatus(int sc)
Sets the status code for this response. This method is used to set the return status code when there is no error (for example, for the status codes SC_OK or SC_MOVED_TEMPORARILY). If there is an error, and the caller wishes to invoke an error-page defined in the web application, the sendError method should be used instead. The container clears the buffer and sets the Location header, preserving cookies and other headers. Parameters: sc - the status code
このメソッドを使う場合はエラーが発生せずに正常にデータが送信される場合に利用します。引数には「HttpServletResponse」インターフェースで定義されてる定数を使用します。一部を抜粋すると次のようなものがあります。
コード | 定数 |
---|---|
200 | SC_OK |
204 | SC_NO_CONTENT |
301 | SC_MOVED_PERMANENTLY |
302 | SC_MOVED_TEMPORARILY |
403 | SC_FORBIDDEN |
404 | SC_NOT_FOUND |
500 | SC_INTERNAL_SERVER_ERROR |
503 | SC_SERVICE_UNAVAILABLE |
ステータスコードは他にも数多く用意されています。200番台は「成功」、300番台は「転送」、400番台は「クライアント側のエラー」、500番台は「サーバ側のエラー」を表します。「setStatus」メソッドは200番台又は300番台のステータスコードを設定する時に利用します。
「setStatus」メソッドを使ってスタータスコードの設定を行った場合、画面に表示する内容は別途作成する必要があります。
エラー系のスタータスコードの設定
エラー系の400番台及び500番台のステータスコードを設定する場合には「HttpServletResponse」インターフェースで定義されている「sendError」メソッドを使います。
sendError public void sendError(int sc) throws java.io.IOException
Sends an error response to the client using the specified status code and clearing the buffer. If the response has already been committed, this method throws an IllegalStateException. After using this method, the response should be considered to be committed and should not be written to. Parameters: sc - the error status code Throws: java.io.IOException - If an input or output exception occurs java.lang.IllegalStateException - If the response was committed before this method call
「sendError」メソッドを使う場合にはスタータスコードの設定を行うと同時に画面に表示される内容を自動で作成します。その為、一般的なエラー表示が画面に行われます。
もし独自のメッセージを画面に表示したい場合には引数の異なる「sendError」メッセージが用意されています。
sendError public void sendError(int sc, java.lang.String msg) throws java.io.IOException
Sends an error response to the client using the specified status. The server defaults to creating the response to look like an HTML-formatted server error page containing the specified message, setting the content type to "text/html", leaving cookies and other headers unmodified. If an error-page declaration has been made for the web application corresponding to the status code passed in, it will be served back in preference to the suggested msg parameter. If the response has already been committed, this method throws an IllegalStateException. After using this method, the response should be considered to be committed and should not be written to. Parameters: sc - the error status code msg - the descriptive message Throws: java.io.IOException - If an input or output exception occurs java.lang.IllegalStateException - If the response was committed
どのように表示されるかはサーブレットコンテナに依存しますが、引数に指定した文字列がエラーページ内に合わせて表示されます。
サンプルプログラム
では簡単なサンプルで試して見ます。別途用意したHTMLフォームから希望するステータスコードを送付すると、それに対応した処理を行います。
フォームが含まれるHTMLページは、次のような簡単なものです。
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html lang="ja"> <head> <meta http-equiv="Content-Type" Content="text/html;charset=Shift_JIS"> <title>サンプル</title> </head> <body> <p>スタータスコードの選択</p> <form action="/sample/ResponseSample3" method="get"> <table> <tr> <td>スタータスコード</td> <td> <select name="code" size="3" multiple> <option value="200">SC_OK</option> <option value="404">SC_NOT_FOUND</option> <option value="500">SC_INTERNAL_SERVER_ERROR</option> </select> </td> </tr> </table> <input type="submit" name="button1" value="送信"> </form> </body> </html>
次にフォームから送られてくるリクエストパラメータを処理するサーブレットを作成します。
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; public class ResponseSample3 extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException{ response.setContentType("text/html; charset=Shift_JIS"); PrintWriter out = response.getWriter(); String tmp; String code = ""; tmp = request.getParameter("code"); if (tmp == null || tmp.length() == 0){ code = "200"; }else{ code = tmp; } if (code.equals("200")){ response.setStatus(HttpServletResponse.SC_OK); StringBuffer sb = new StringBuffer(); sb.append("<html>"); sb.append("<head>"); sb.append("<title>サンプル</title>"); sb.append("</head>"); sb.append("<body>"); sb.append("<p>200:SC_OK</p>"); sb.append("</body>"); sb.append("</html>"); out.println(new String(sb)); out.close(); }else{ if (code.equals("404")){ response.sendError(HttpServletResponse.SC_NOT_FOUND, "見つかりません"); }else if (code.equals("500")){ response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR); } } } }
サンプルプログラムをコンパイルして作成した「ResponseSample3.class」ファイルを別途作成した「web.xml」ファイルを次のように配置します。
D:\ -- servlet-sample | +-- WEB-INF | +-- (formsample5.html) | +-- (web.xml) | +-- classes | +-- (ResponseSample3.class)
web.xmlファイルは次のようになります。
<?xml version="1.0" encoding="ISO-8859-1"?> <web-app xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd" version="2.4"> <servlet> <servlet-name>ResponseSample3</servlet-name> <servlet-class>ResponseSample3</servlet-class> </servlet> <servlet-mapping> <servlet-name>ResponseSample3</servlet-name> <url-pattern>/ResponseSample3</url-pattern> </servlet-mapping> </web-app>
コンテキストファイルを作成し「(Tomcatをインストールしたディレクトリ)\Tomcat 5.5\conf\Catalina\localhost\」ディレクトリに「sample.xml」ファイルとして保存します。内容は以下の通りです。
<Context path="/sample" docBase="d:/servlet-sample/sample"> </Context>
準備は以上です。ではTomcatを再起動してから「http://localhost:8080/sample/formsample5.html」へブラウザでアクセスして下さい。
フォームが表示されます。まず「SC_OK」を選択してから「送信」ボタンをクリックして下さい。
この場合は「setStatus」メソッドを使ってステータスコードを設定しています。画面に表示される内容は自分で出力したものになります。
では元のフォーム画面に戻って今度は「SC_NOT_FOUND」を選択してから「送信」ボタンをクリックします。
今度はTomcat側でエラー画面を自動で作成してくれます。基本的には通常Tomcatが出力するエラー画面と同じです。今回は別途表示メッセージを指定しているため合わせて表示されています。
( Written by Tatsuo Ikura )
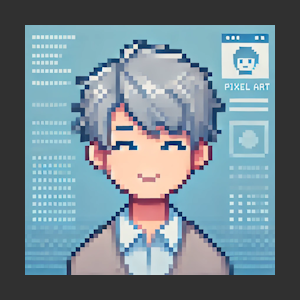
著者 / TATSUO IKURA
これから IT 関連の知識を学ばれる方を対象に、色々な言語でのプログラミング方法や関連する技術、開発環境構築などに関する解説サイトを運営しています。