- Home ›
- サーブレット/JSP入門 ›
- ユーザー認証
認証された情報の取得
認証が通った後に表示されるサーブレットなどから、認証されたユーザーに関する情報を取得することができます。
情報を取得するためにHttpServletRequestインターフェースでいくつかのメソッドが定義されています。
getRemoteUserメソッド
「getRemoteUser」メソッドは認証が行われたユーザー名を取得します。
getRemoteUser public java.lang.String getRemoteUser()
Returns the login of the user making this request, if the user has been authenticated, or null if the user has not been authenticated. Whether the user name is sent with each subsequent request depends on the browser and type of authentication. Same as the value of the CGI variable REMOTE_USER. Returns: a String specifying the login of the user making this request, or null if the user login is not known
戻り値として認証に成功したユーザー名が帰ってきます。認証が行われていなければnullが帰ってきます。
getAuthTypeメソッド
「getAuthType」メソッドは認証がどの方式で行われたのかを取得します。
getAuthType public java.lang.String getAuthType()
Returns the name of the authentication scheme used to protect the servlet. All servlet containers support basic, form and client certificate authentication, and may additionally support digest authentication. If the servlet is not authenticated null is returned. Same as the value of the CGI variable AUTH_TYPE. Returns: one of the static members BASIC_AUTH, FORM_AUTH, CLIENT_CERT_AUTH, DIGEST_AUTH (suitable for == comparison) or the container-specific string indicating the authentication scheme, or null if the request was not authenticated.
戻り値として認証方式を表すBASIC、FORM、CLIENT_CERT、DIGESTのどれか1つの値が帰ってきます。認証が行われていなければnullが帰ってきます。
isUserInRoleメソッド
「isUserInRole」メソッドは認証されたユーザーが引数に指定したロールに属しているかどうかを判別してくれます。複数のロールに対してアクセスを許可していた場合に、どのロールで許可されたのかを判別して処理を分けたい場合などに便利です。
isUserInRole public boolean isUserInRole(java.lang.String role)
Returns a boolean indicating whether the authenticated user is included in the specified logical "role". Roles and role membership can be defined using deployment descriptors. If the user has not been authenticated, the method returns false. Parameters: role - a String specifying the name of the role Returns: a boolean indicating whether the user making this request belongs to a given role; false if the user has not been authenticated
引数に判別したいロールを文字列で指定します。認証に成功したユーザーがロールに属していれば「true」が帰ってきます。
getUserPrincipaメソッド
「getUserPrincipa」メソッドは「java.security.Principal」インターフェースを実装したオブジェクトを取得できます。
getUserPrincipal public java.security.Principal getUserPrincipal()
Returns a java.security.Principal object containing the name of the current authenticated user. If the user has not been authenticated, the method returns null. Returns: a java.security.Principal containing the name of the user making this request; null if the user has not been authenticated
このオブジェクトは主体という抽象的な概念を表すようですが、このオブジェクトからさらに「getName」メソッドでユーザー名を取得する事ができます。結果的に「getRemoteUser」メソッドと同じ結果が取得できます。
getName String getName()
主体の名前を返します。 戻り値: この主体の名前
「getRemoteUser」メソッドとの使い分けについては良く分かっていません。
サンプルプログラムの修正
それではユーザー認証の対象となっているサーブレットに対して、認証が通ったユーザーに関する情報を表示するように変更してみます。
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; public class AuthTest3 extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException{ response.setContentType("text/html; charset=Shift_JIS"); PrintWriter out = response.getWriter(); out.println("<html>"); out.println("<head>"); out.println("<title>ユーザー認証テスト</title>"); out.println("</head>"); out.println("<body>"); out.println("<p>User Name : "); out.println(request.getRemoteUser()); out.println("</p>"); out.println("<p>Principal Name : "); out.println(request.getUserPrincipal().getName()); out.println("</p>"); out.println("<p>Auth Type : "); out.println(request.getAuthType()); out.println("</p>"); out.println("<p>Sales Div? : "); out.println(request.isUserInRole("sales")); out.println("</p>"); out.println("</body>"); out.println("</html>"); } }
レルムはJDBCRealmレルムを使います。
<Realm className="org.apache.catalina.realm.JDBCRealm" driverName="com.mysql.jdbc.Driver" connectionURL="jdbc:mysql://localhost/auth" connectionName="authtest" connectionPassword="authtest" userTable="user_table" userNameCol="user" userCredCol="pass" userRoleTable="role_table" roleNameCol="role" />
MySQLの設定についてはBASIC認証の時と同じ設定を使います。詳しくは『BASIC認証(JDBCRealmレルム)』を参照して下さい。
次にweb.xmlファイルを次の条件で作成します。今回はDIGEST認証を使います。
リソース名:User Digest Auth 対象サーブレット:このアプリケーションに含まれる全てのサーブレット 対象のHTTPメソッド:全て 許可するロール名:sales 認証方式:DIGEST認証 レルム名:User Digest Auth
作成したweb.xmlファイルは次の通りです。
<?xml version="1.0" encoding="ISO-8859-1"?> <web-app xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd" version="2.4"> <servlet> <servlet-name>AuthTest3</servlet-name> <servlet-class>AuthTest3</servlet-class> </servlet> <servlet-mapping> <servlet-name>AuthTest3</servlet-name> <url-pattern>/AuthTest3</url-pattern> </servlet-mapping> <security-constraint> <web-resource-collection> <web-resource-name>User Digest Auth</web-resource-name> <url-pattern>/*</url-pattern> </web-resource-collection> <auth-constraint> <role-name>sales</role-name> </auth-constraint> </security-constraint> <login-config> <auth-method>DIGEST</auth-method> <realm-name>User Digest Auth</realm-name> </login-config> <security-role> <role-name>sales</role-name> </security-role> </web-app>
それでは一度サーブレットを再起動してから、ブラウザで「http://localhost:8080/auth/AuthTest3」へアクセスして下さい。
上記のようにログイン用のページが表示されました。ここでデータベースに登録したユーザー名「itou」パスワード「itou」を入力します。
「itou」はユーザー認証が通り、所属するロールがアクセス許可に設定してるため、ページを見ることができます。
ユーザー認証に成功したユーザー名、認証方式、そして認証されたユーザーが「sales」ロールかどうかの判定結果が表示されています。
( Written by Tatsuo Ikura )
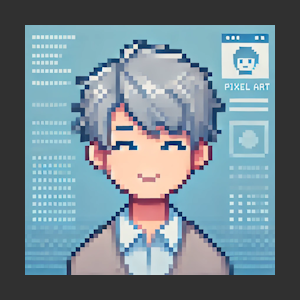
著者 / TATSUO IKURA
これから IT 関連の知識を学ばれる方を対象に、色々な言語でのプログラミング方法や関連する技術、開発環境構築などに関する解説サイトを運営しています。